Top 10 JavaScript Utility Functions to Enhance Your Coding Skills
Written on
Chapter 1: Overview of JavaScript Utility Functions
In this article, we will delve into the ten most useful utility functions in JavaScript, showcasing practical examples to illustrate their applications. Mastering these functions will enable you to write cleaner and more efficient code.
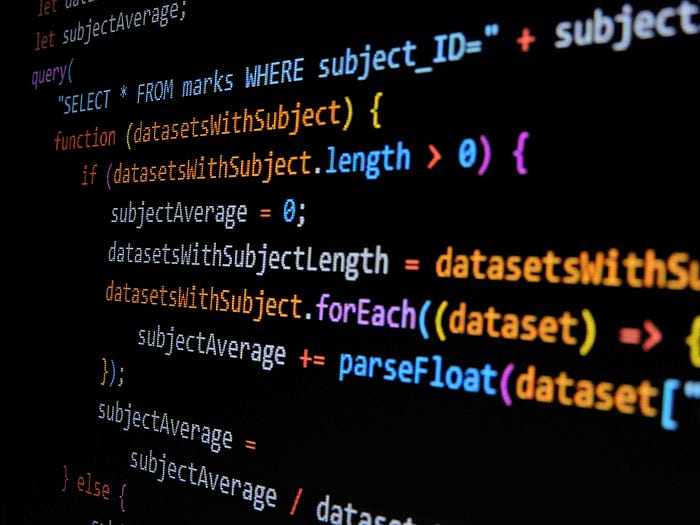
Section 1.1: Transforming Arrays with Map
The map function is a powerful tool for transforming arrays. It applies a specified function to each element, generating a new array that contains the modified values.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map((num) => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
Section 1.2: Filtering Arrays with Filter
The filter method allows you to create a new array that includes only those elements meeting a particular condition.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
Subsection 1.2.1: Reducing Arrays with Reduce
The reduce function condenses an array into a single value by applying a function that aggregates the results.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum); // Output: 15
Section 1.3: Iterating with forEach
The forEach method executes a provided function for each element in an array, making it ideal for side effects.
const colors = ['red', 'green', 'blue'];
colors.forEach((color) => console.log(color));
// Output:
// red
// green
// blue
Section 1.4: Finding Elements with Find
The find method retrieves the first element in an array that meets a specific condition.
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
];
const user = users.find((user) => user.id === 2);
console.log(user); // Output: { id: 2, name: 'Bob' }
Section 1.5: Locating Element Index with indexOf
The indexOf function returns the index of the first occurrence of a specified item in an array, returning -1 if not found.
const fruits = ['apple', 'banana', 'cherry'];
const index = fruits.indexOf('banana');
console.log(index); // Output: 1
Section 1.6: Checking Conditions with some
The some method verifies if at least one element in an array satisfies a given condition, returning a boolean.
const numbers = [1, 3, 5, 7, 8];
const hasEven = numbers.some((num) => num % 2 === 0);
console.log(hasEven); // Output: true
Section 1.7: Validating Conditions with every
The every function checks if all elements in an array meet a specified condition, returning true or false.
const ages = [25, 30, 35, 40];
const overTwenty = ages.every((age) => age > 20);
console.log(overTwenty); // Output: true
Section 1.8: Sorting Arrays with sort
The sort method arranges the elements of an array in ascending or descending order based on a comparison function.
const fruits = ['banana', 'apple', 'cherry'];
fruits.sort();
console.log(fruits); // Output: ['apple', 'banana', 'cherry']
Section 1.9: Merging Arrays with concat
The concat method combines two or more arrays into a new one containing all the elements.
const fruits = ['apple', 'banana'];
const vegetables = ['carrot', 'broccoli'];
const combined = fruits.concat(vegetables);
console.log(combined); // Output: ['apple', 'banana', 'carrot', 'broccoli']
Chapter 2: Practical Applications
In this section, we will further examine the practical applications of these utility functions, enhancing your JavaScript skills.
Explore the "JavaScript Array Cardio Practice - Day 1" video to see these functions in action and improve your understanding of array manipulations.
Additionally, check out the "Utility Maximization Problem with Linear Utilities" video for insights into problem-solving strategies that can be applied in programming.
In Summary 🌟
In this article, we have examined ten essential utility functions in JavaScript that can greatly simplify your coding tasks. By understanding and utilizing these functions, you can enhance your programming skills, making your code both more efficient and readable.
Thank you for joining us! Don't forget to follow and engage with our community on various platforms for more insights and content.