Getting Started with React Suite for Step Displays in React
Written on
Chapter 1: Introduction to React Suite
React Suite is a powerful UI library that simplifies the process of integrating various components into your React application. This guide will explore how to utilize this library for displaying steps effectively in your app.
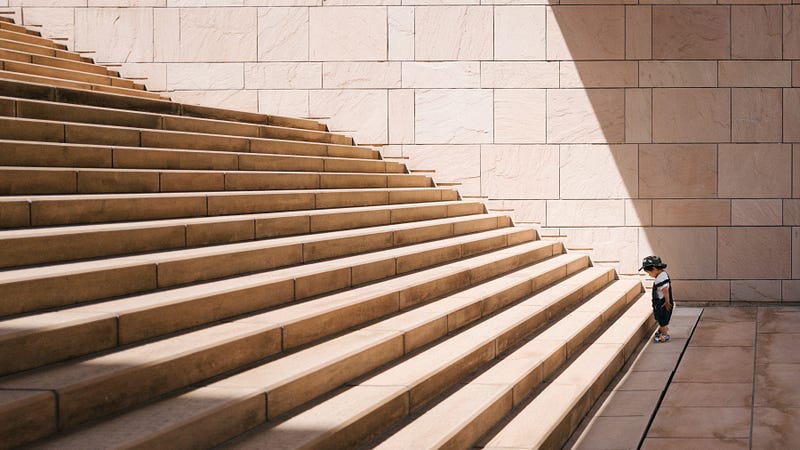
Section 1.1: Implementing the Steps Component
To display steps, we can leverage the Steps component from React Suite. Below is an example code snippet demonstrating how to set it up:
import React from "react";
import { Steps } from "rsuite";
import "rsuite/dist/styles/rsuite-default.css";
export default function App() {
return (
<div className="App">
<Steps current={1}>
<Steps.Item />
<Steps.Item />
<Steps.Item />
<Steps.Item />
</Steps>
</div>
);
}
In this example, the current property is used to specify the index of the active step.
Section 1.2: Customizing Steps with Titles and Descriptions
You can enhance the Steps component by adding titles and descriptions for each step. Here’s how you can do it:
import React from "react";
import { Steps } from "rsuite";
import "rsuite/dist/styles/rsuite-default.css";
export default function App() {
return (
<div className="App">
<Steps current={1}>
<Steps.Item title="Finished" description="This is a description." />
<Steps.Item title="In Progress" description="This is a description." />
<Steps.Item title="Waiting" description="This is a description." />
</Steps>
</div>
);
}
Chapter 2: Advanced Features of the Steps Component
The first video titled "React Suite Introduction" provides a comprehensive overview of the React Suite library and its components.
You can also modify the layout to display steps vertically by using the vertical property:
import React from "react";
import { Steps } from "rsuite";
import "rsuite/dist/styles/rsuite-default.css";
export default function App() {
return (
<div className="App">
<Steps current={1} vertical>
<Steps.Item title="Finished" description="This is a description." />
<Steps.Item title="In Progress" description="This is a description." />
<Steps.Item title="Waiting" description="This is a description." />
</Steps>
</div>
);
}
To change the color of the current step's status, utilize the currentStatus property:
import React from "react";
import { Steps } from "rsuite";
import "rsuite/dist/styles/rsuite-default.css";
export default function App() {
return (
<div className="App">
<Steps current={1} currentStatus="error">
<Steps.Item title="Finished" description="This is a description." />
<Steps.Item title="In Progress" description="This is a description." />
<Steps.Item title="Waiting" description="This is a description." />
</Steps>
</div>
);
}
For a more compact display, you can make the steps smaller by using the small property:
import React from "react";
import { Steps } from "rsuite";
import "rsuite/dist/styles/rsuite-default.css";
export default function App() {
return (
<div className="App">
<Steps current={1} small>
<Steps.Item title="Finished" />
<Steps.Item title="In Progress" />
<Steps.Item title="Waiting" />
</Steps>
</div>
);
}
Additionally, you can incorporate icons into your steps using the icon property:
import React from "react";
import { Steps, Icon } from "rsuite";
import "rsuite/dist/styles/rsuite-default.css";
export default function App() {
return (
<div className="App">
<Steps current={1}>
<Steps.Item
title="Finished"
icon={<Icon icon="pencil-square" size="lg" />}
/>
<Steps.Item title="In Progress" icon={<Icon icon="book" size="lg" />} />
<Steps.Item title="Waiting" icon={<Icon icon="wechat" size="lg" />} />
</Steps>
</div>
);
}
The second video titled "React JS Full Course for Beginners" offers a complete guide to mastering React, including the use of libraries like React Suite.
Conclusion
Incorporating a step display into your React application can be easily achieved using React Suite. This library provides numerous customization options to enhance user experience.