Converting Date Strings to Timestamps in JavaScript
Written on
Chapter 1: Introduction to Timestamps
When working with JavaScript, you may often need to convert date strings into UNIX timestamps. This guide will walk you through different methods to achieve this conversion effectively.
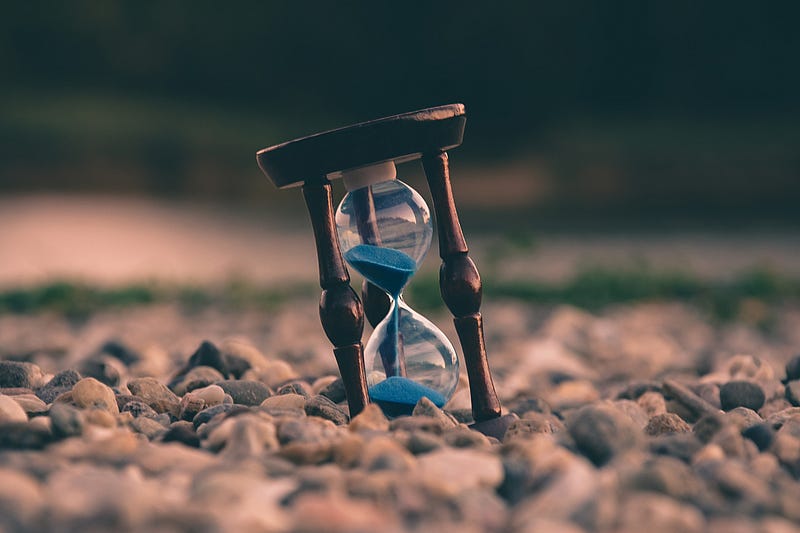
Photo by Aron Visuals on Unsplash
Section 1.1: Using the Date.parse() Method
A straightforward approach to convert a date string to a timestamp is by utilizing the Date.parse() method. Below is an example of how to implement this:
const toTimestamp = (strDate) => {
const dt = Date.parse(strDate);
return dt / 1000;
}
console.log(toTimestamp('02/13/2020 23:31:30'));
In this example, the toTimestamp() function leverages Date.parse() to interpret the date string and convert it into a timestamp. Since the result is in milliseconds, we divide by 1000 to get the equivalent in seconds.
Section 1.2: Utilizing the getTime() Method
Another effective method is to use the getTime() function associated with a Date object. Here’s how you can do this:
const toTimestamp = (strDate) => {
const dt = new Date(strDate).getTime();
return dt / 1000;
}
console.log(toTimestamp('02/13/2020 23:31:30'));
In this case, we create a new Date instance using the provided string and invoke getTime() to retrieve the timestamp in milliseconds, which we again convert to seconds by dividing by 1000.
Subsection 1.2.1: Converting Using Moment.js
Moment.js offers a convenient way to convert date strings into timestamps through its unix() method. Here is an example:
const toTimestamp = (strDate) => {
const dt = moment(strDate).unix();
return dt;
}
console.log(toTimestamp('02/13/2020 23:31:30'));
In this example, the strDate is passed to the moment() function, which generates a moment object. The unix() method is then called to return the timestamp, conveniently provided in seconds, eliminating the need for further conversion.
Chapter 2: Conclusion
In summary, both vanilla JavaScript and Moment.js provide effective methods for converting date strings into UNIX timestamps. Whether you prefer using built-in functions or an external library, you have multiple options at your disposal.
Learn how to convert timestamps to date formats effectively in this informative video.
Explore how to convert Firestore timestamps to JavaScript date objects in this helpful tutorial.